Coding 6: Conditional Expressions, and IF Else Statements.
We'll talk about some of conditional expressions, and symbols you need to know, and how: IF, ELIF, and ELSE work.
Quick Recap
Welcome back, as of now you all know how to create loops in order to automate tedious work. You also know how to create your own custom functions so that you can start to specialize in whatever direction you choose. For those interested in Cybersecurity, and Python, check out: BowTiedCyber.
Table of Contents:
Conditional Expressions
Gates (AND/OR/NOT)
IF/ELIF/ELSE Statements
1 - Conditional Expressions
When we are coding, sometimes instead of giving us a string, or a number as an answer, sometimes we would rather the computer do a lot of work for us, and tell us if something is True or False. We already had a taste of this with our startsWith, and endsWith functions. Now, we will talk about all sort of different expressions you can create that will help check for something, and give us a True or False.
The True and False answers that we get are referred to as a Boolean data type.
You can use this table below which shows you how to use logical operators:
Python
Here is an example of using Python to check if 4 is not the same thing as 5.
R
Here is an example of using R to check if 4 is not the same thing as 5.
2 - Gates (AND/OR/NOT)
Using the above table you can see that we can using Python/R in order to test if a condition is true or false. Now, we will introduce a concept of using gates to combine multiple conditional (Boolean) expressions together.
And
There is something called an and keyword, we can use it to combine 2 boolean values together. For example, we can combine True and True together, and if we look at the first row, we can see that True and True evaluates to True, and all other cases evaluate to False.
OR
There is something called an or keyword, once again, we can use it to combine 2 Boolean values together. From the above table, you can see that False or False is the only way to evaluate to a False, all other scenarios would give us True.
NOT
This is the late logic gate (keyword) we will touch upon. This one is called the not keyword. All it does is flip from True to False, and vice versa.
Python
We will run a few simple examples of using the 3 keywords from above.
R
In R, we do not spell out the words True, False, and, etc… But instead we use the following for mapping:
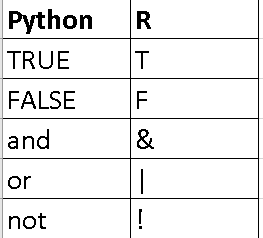
3 - IF/ELIF/ELSE Statements
I think at this point everyone has basically already seen what if else statements, and logic looks like. But, here is a quick visual on how it works:
Basically, it will check your If statement first, if it is False, then it will check the elifs one by one, if they are also False, then it will automatically execute the else statement. Remember, coding in R, and Python works top down, so the moment the if/elif/else block runs into a True, it immediately executes the statement, and ignores everything else.
Python
Here is a simple if, elif, and else statement.
R
R doesn’t use the word elif, instead it uses else if as a combination to re-create elif.
Note: Next post will be on setting up the: numpy, pandas, data.table, and the h2o libraries by using pip, and RStudio. We are about to start the real meat of Data Science now.
Click here to continue to coding 7: which is all about libraries.